Card
Cards are a popular and flexible UI component.
Basics
A card is just an element with a .card
class applied. You can put any kind of content inside.
Make sure you wrap your content in a .card-section
element in order to achieve the traditional card look.
A card container has no padding, allowing you to place full-bleed images inside. Use the .card-divider
and .card-section
classes to sub-divide a card.
The .card
and .card-divider
elements are flexbox containers. This allows you to use Flexbox Utilities to create more flexible layouts.
<div class="card" style="width: 300px;">
<div class="card-divider">
This is a header
</div>
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style, and is appropriately subdued.</p>
</div>
</div>
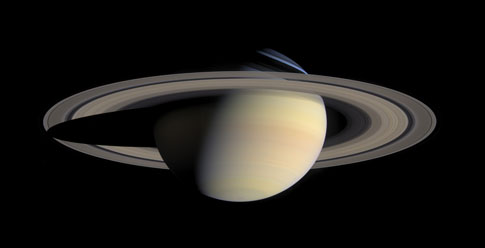
This is a card.
It has an easy to override visual style, and is appropriately subdued.
Browser Bug (IE 11 - Flexbug): On IE 11, when using the card with image, you may see a lot of whitespace under each image that happens to match the original image size. The bug can be reproduced here on an IE11 browser. Use .card-image
class to wrap your image to resolve this.
<div class="card-image">
<img src="assets/img/generic/rectangle-1.jpg">
</div>
Card Divider
You can also include a .card-divider
element as a title, footer or to break up content.
<div class="card" style="width: 300px;">
<div class="card-divider">
<h4>I'm featured</h4>
</div>
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<p>This card makes use of the card-divider element.</p>
</div>
</div>
I'm featured
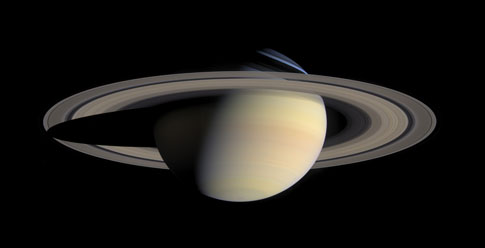
This card makes use of the card-divider element.
Images
Images play nicely with cards. Simply include one outside of the .card-section
element to span nicely to the edges. Or move the image inside the .card-section
to have padding around the image.
<!-- image has no padding -->
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<p>This is a simple card with an image.</p>
</div>
</div>
<!-- image has padding -->
<div class="card">
<div class="card-section">
<img src="assets/img/generic/rectangle-1.jpg">
</div>
<div class="card-section">
<p>This is a simple card with an image inside a `.card-section`.</p>
</div>
</div>
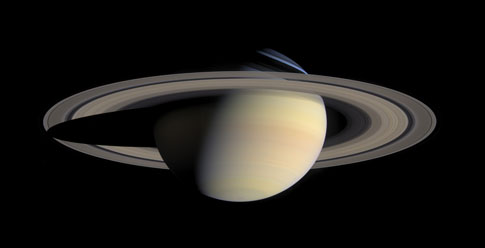
This is a simple card with an image.
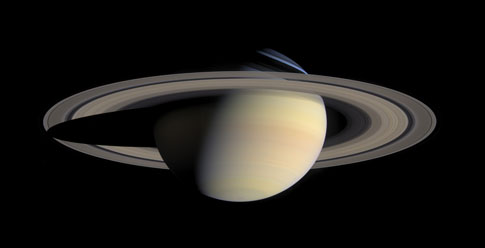
This is a simple card with an image inside a `.card-section`.
<div class="card">
<div class="card-section">
<p>Images work just fine below the content too!</p>
</div>
<img src="assets/img/generic/rectangle-1.jpg">
</div>
Images work just fine below the content too!
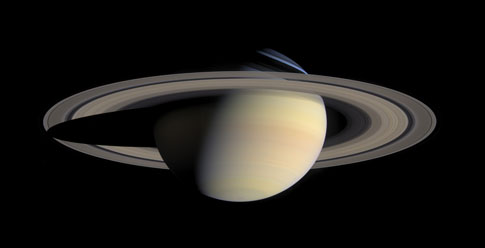
Sizing
You can either set the width of cards with custom css or add them into the Foundation grid.
<div class="grid-container">
<div class="grid-x grid-margin-x small-up-2 medium-up-3">
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a row of cards.</h4>
<p>This row of cards is embedded in an X-Y Block Grid.</p>
</div>
</div>
</div>
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style, and is appropriately subdued.</p>
</div>
</div>
</div>
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style, and is appropriately subdued.</p>
</div>
</div>
</div>
</div>
</div>
<div class="grid-x grid-margin-x small-up-2 medium-up-3">
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a row of cards.</h4>
<p>This row of cards is embedded in an Flex Block Grid.</p>
</div>
</div>
</div>
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style, and is appropriately subdued.</p>
</div>
</div>
</div>
<div class="cell">
<div class="card">
<img src="assets/img/generic/rectangle-1.jpg">
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style, and is appropriately subdued.</p>
</div>
</div>
</div>
</div>
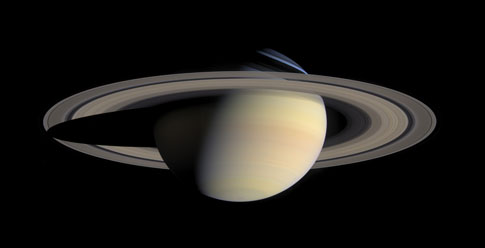
This is a row of cards.
This row of cards is embedded in an X-Y Block Grid.
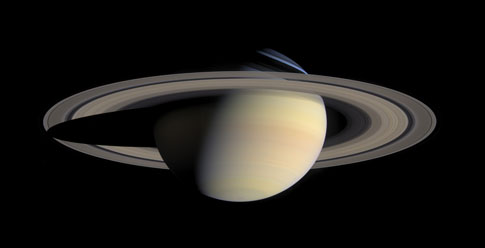
This is a card.
It has an easy to override visual style, and is appropriately subdued.
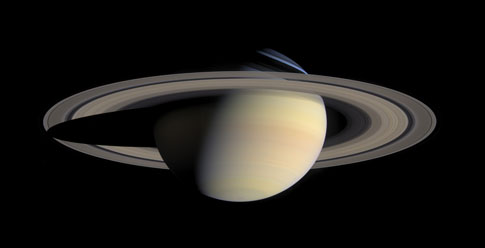
This is a card.
It has an easy to override visual style, and is appropriately subdued.
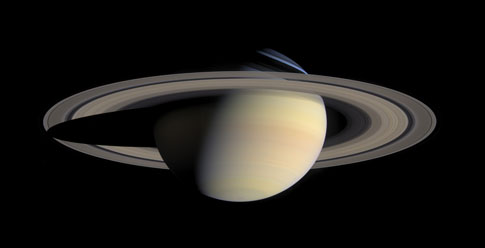
This is a row of cards.
This row of cards is embedded in an Flex Block Grid.
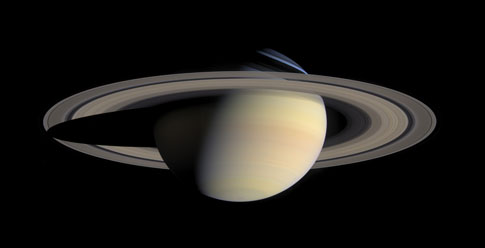
This is a card.
It has an easy to override visual style, and is appropriately subdued.
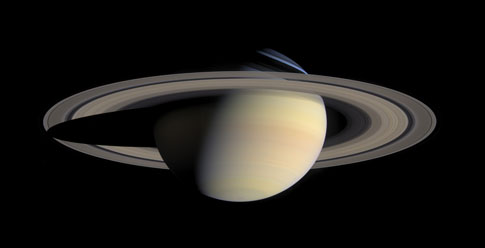
This is a card.
It has an easy to override visual style, and is appropriately subdued.
Sass Reference
Variables
The default styles of this component can be customized using these Sass variables in your project's settings file.
Name | Type | Default Value | Description |
---|---|---|---|
$card-background |
Color | $white |
Default background color. |
$card-font-color |
Color | $body-font-color |
Default font color for cards. |
$card-divider-background |
Color | $light-gray |
Default background. |
$card-border |
List | 1px solid $light-gray |
Default border style. |
$card-shadow |
List | none |
Default card shadow. |
$card-border-radius |
List | $global-radius |
Default border radius. |
$card-padding |
Number | $global-padding |
Default padding. |
$card-margin-bottom |
number | $global-margin |
Default bottom margin. |
Mixins
We use these mixins to build the final CSS output of this component. You can use the mixins yourself to build your own class structure out of our components.
card-container
@include card-container($background, $color, $margin, $border, $radius, $shadow);
Adds styles for a card container.
Parameter | Type | Default Value | Description |
---|---|---|---|
$background |
Color | None |
Background color of the card. |
$color |
Color | None |
font color of the card. |
$margin |
Number | None |
Bottom margin of the card. |
$border |
List | None |
Border around the card. |
$radius |
List | None |
border radius of the card. |
$shadow |
List | None |
box shadow of the card. |
card-divider
@include card-divider;
Adds styles for a card divider.
card-section
@include card-section;
Adds styles for a card section.