Tabs
JavaScriptTabs are elements that help you organize and navigate multiple documents in a single container. They can be used for switching between items in the container.
Basics
There are two pieces to a tabbed interface: the tabs themselves, and the content for each tab. The tabs are an element with the class .tabs
, and each item has the class .tabs-title
. Each tab contains a link to a tab. The href
of each link should match the ID of a tab. Alternatively, the ID can be specified with the attribute data-tabs-target
.
<ul class="tabs" data-tabs id="example-tabs">
<li class="tabs-title is-active"><a href="#panel1" aria-selected="true">Tab 1</a></li>
<li class="tabs-title"><a data-tabs-target="panel2" href="#panel2">Tab 2</a></li>
</ul>
The tab content container has the class .tabs-content
, while each section has the class .tabs-panel
. Each content pane also has a unique ID, which is targeted by a link in the tabstrip.
<div class="tabs-content" data-tabs-content="example-tabs">
<div class="tabs-panel is-active" id="panel1">
<p>Vivamus hendrerit arcu sed erat molestie vehicula. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Suspendisse dictum feugiat nisl ut dapibus.</p>
</div>
<div class="tabs-panel" id="panel2">
<p>Suspendisse dictum feugiat nisl ut dapibus. Vivamus hendrerit arcu sed erat molestie vehicula. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor.</p>
</div>
</div>
Put it all together, and we get this:
two
three
Check me out! I'm a super cool Tab panel with text content!
four
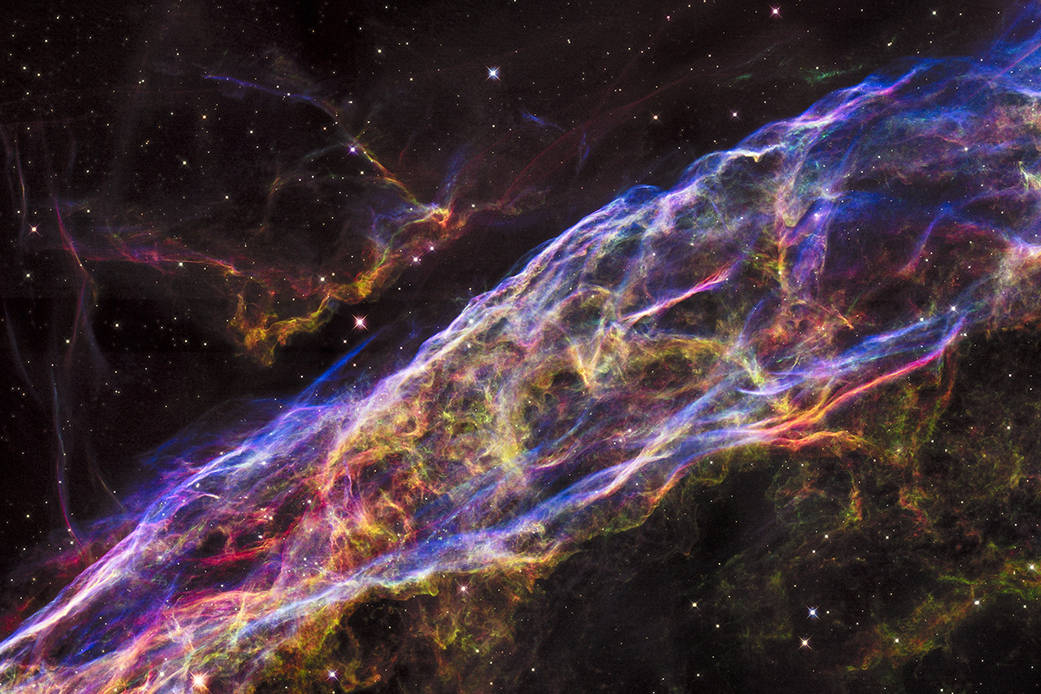
five
Check me out! I'm a super cool Tab panel with text content!
six
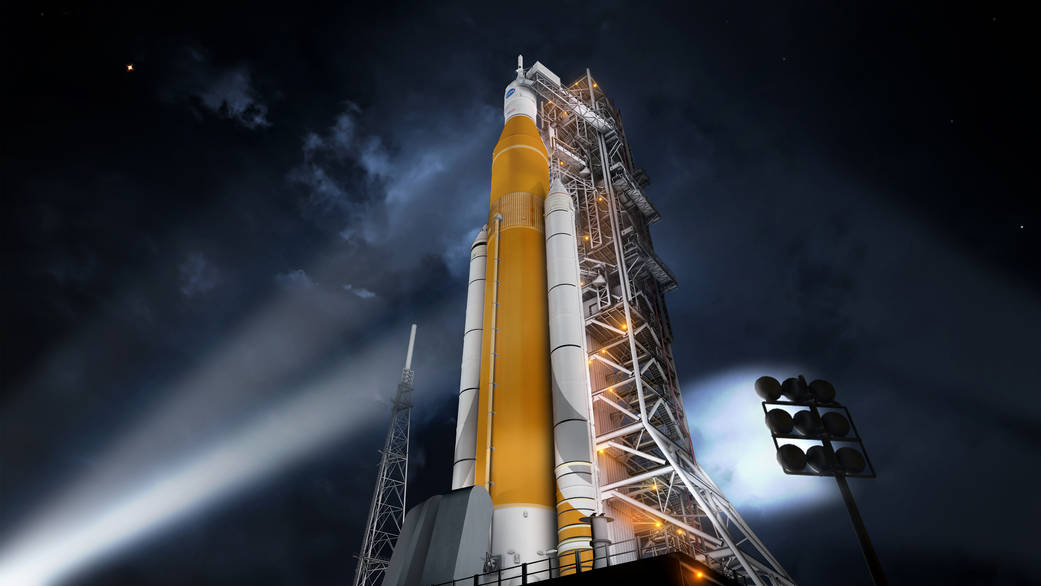
Vertical Tabs
Add the .vertical
class to a tabstrip and tab contents to stack tabs vertically. You can also remove the .grid-margin-x
class from the wrapping div to make them sit side-by-side.
<div class="grid-container">
<div class="grid-x">
<div class="cell medium-3">
<ul class="vertical tabs" data-tabs id="example-tabs">
<li class="tabs-title is-active"><a href="#panel1v" aria-selected="true">Tab 1</a></li>
<li class="tabs-title"><a href="#panel2v">Tab 2</a></li>
<li class="tabs-title"><a href="#panel3v">Tab 3</a></li>
<li class="tabs-title"><a href="#panel4v">Tab 4</a></li>
<li class="tabs-title"><a href="#panel5v">Tab 5</a></li>
<li class="tabs-title"><a href="#panel6v">Tab 6</a></li>
</ul>
</div>
<div class="cell medium-9">
<div class="tabs-content vertical" data-tabs-content="example-tabs">
<div class="tabs-panel is-active" id="panel1v">
<p>One</p>
<p>Check me out! I'm a super cool Tab panel with text content!</p>
</div>
<div class="tabs-panel" id="panel2v">
<p>Two</p>
<img class="thumbnail" src="assets/img/generic/rectangle-7.jpg">
</div>
<div class="tabs-panel" id="panel3v">
<p>Three</p>
<p>Check me out! I'm a super cool Tab panel with text content!</p>
</div>
<div class="tabs-panel" id="panel4v">
<p>Four</p>
<img class="thumbnail" src="assets/img/generic/rectangle-2.jpg">
</div>
<div class="tabs-panel" id="panel5v">
<p>Five</p>
<p>Check me out! I'm a super cool Tab panel with text content!</p>
</div>
<div class="tabs-panel" id="panel6v">
<p>Six</p>
<img class="thumbnail" src="assets/img/generic/rectangle-8.jpg">
</div>
</div>
</div>
</div>
</div>
Collapsing Tabs
Add the attribute data-active-collapse="true"
to a tabstrip to collapse active tabs.
<ul class="tabs" data-active-collapse="true" data-tabs id="collapsing-tabs">
<li class="tabs-title is-active"><a href="#panel1c" aria-selected="true">Tab 1</a></li>
<li class="tabs-title"><a href="#panel2c">Tab 2</a></li>
<li class="tabs-title"><a href="#panel3c">Tab 3</a></li>
<li class="tabs-title"><a href="#panel4c">Tab 4</a></li>
</ul>
<div class="tabs-content" data-tabs-content="collapsing-tabs">
<div class="tabs-panel is-active" id="panel1c">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
<div class="tabs-panel" id="panel2c">
<p>Vivamus hendrerit arcu sed erat molestie vehicula. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Suspendisse dictum feugiat nisl ut dapibus.</p>
</div>
<div class="tabs-panel" id="panel3c">
<img class="thumbnail" src="assets/img/generic/rectangle-3.jpg">
</div>
<div class="tabs-panel" id="panel4c">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
</div>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Vivamus hendrerit arcu sed erat molestie vehicula. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Suspendisse dictum feugiat nisl ut dapibus.
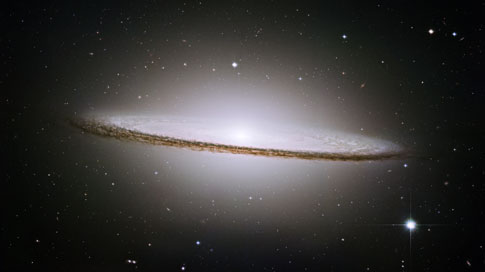
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Tabs and URLs
Browser history
When the data-deep-link
option is set to true
, the current state of the tabset is recorded by adding a hash with the tab panel ID to the browser URL when a tab opens. By default, tabs replace the browser history (using history.replaceState()
). Modify this behavior by using attribute data-update-history="true"
to append to the browser history (using history.pushState()
). In the latter case the browser back button will track each click that opens a tab panel.
By using deep linking (see below), the open state of a page's tabset may be shared by copy-pasting the browser URL.
Deep linking
Add the attribute data-deep-link="true"
to a tabstrip to:
- modify the browser history when a tab is clicked
- allow users to open a particular tab at page load with a hash-appended URL
<ul class="tabs" data-deep-link="true" data-update-history="true" data-deep-link-smudge="true" data-deep-link-smudge-delay="500" data-tabs id="deeplinked-tabs">
<li class="tabs-title is-active"><a href="#panel1d" aria-selected="true">Tab 1</a></li>
<li class="tabs-title"><a href="#panel2d">Tab 2</a></li>
<li class="tabs-title"><a href="#panel3d">Tab 3</a></li>
<li class="tabs-title"><a href="#panel4d">Tab 4</a></li>
</ul>
<div class="tabs-content" data-tabs-content="deeplinked-tabs">
<div class="tabs-panel is-active" id="panel1d">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
<div class="tabs-panel" id="panel2d">
<p>Vivamus hendrerit arcu sed erat molestie vehicula. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Suspendisse dictum feugiat nisl ut dapibus.</p>
</div>
<div class="tabs-panel" id="panel3d">
<img class="thumbnail" src="assets/img/generic/rectangle-3.jpg">
</div>
<div class="tabs-panel" id="panel4d">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
</div>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Vivamus hendrerit arcu sed erat molestie vehicula. Sed auctor neque eu tellus rhoncus ut eleifend nibh porttitor. Ut in nulla enim. Phasellus molestie magna non est bibendum non venenatis nisl tempor. Suspendisse dictum feugiat nisl ut dapibus.
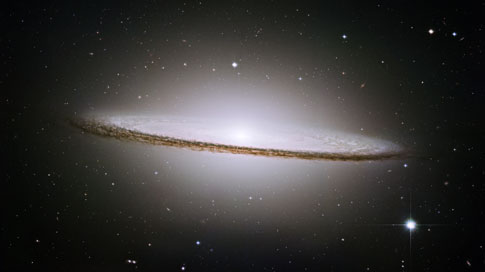
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
For example, https://example.com/#panel3d will open the third tab panel at page load. This example will open a new browser tab and scroll you to the open tab.
When linking directly to a tab panel, it might not be obvious that the content appears within a tab panel. An additional attribute data-deep-link-smudge
rolls the page up slightly after deep linking (to a horizontal tabset) so that the tabstrip is at the top of the viewport.
<ul class="tabs" data-deep-link="true" data-deep-link-smudge="true" data-deep-link-smudge-delay="600" data-tabs id="deeplinked-tabs-with-smudge">
Sass Reference
Variables
The default styles of this component can be customized using these Sass variables in your project's settings file.
Name | Type | Default Value | Description |
---|---|---|---|
$tab-margin |
Number | 0 |
Default margin of the tab bar. |
$tab-background |
Color | $white |
Default background color of a tab bar. |
$tab-color |
Color | $primary-color |
Font color of tab item. |
$tab-background-active |
Color | $light-gray |
Active background color of a tab bar. |
$tab-active-color |
Color | $primary-color |
Active font color of tab item. |
$tab-item-font-size |
Number | rem-calc(12) |
Font size of tab items. |
$tab-item-background-hover |
$white |
Default background color on hover for items in a Menu. |
|
$tab-item-padding |
Number | 1.25rem 1.5rem |
Default padding of a tab item. |
$tab-content-background |
Color | $white |
Default background color of tab content. |
$tab-content-border |
Color | $light-gray |
Default border color of tab content. |
$tab-content-color |
Color | $body-font-color |
Default text color of tab content. |
$tab-content-padding |
Number or List | 1rem |
Default padding for tab content. |
Mixins
We use these mixins to build the final CSS output of this component. You can use the mixins yourself to build your own class structure out of our components.
tabs-container
@include tabs-container;
Adds styles for a tab container. Apply this to a <ul>
.
tabs-container-vertical
@include tabs-container-vertical;
Augments a tab container to have vertical tabs. Use this in conjunction with tabs-container()
.
tabs-title
@include tabs-title;
Adds styles for the links within a tab container. Apply this to the <li>
elements inside a tab container.
tabs-content
@include tabs-content;
Adds styles for the wrapper that surrounds a tab group's content panes.
tabs-content-vertical
@include tabs-content-vertical;
Augments a tab content container to have a vertical style, by shifting the border around. Use this in conjunction with tabs-content()
.
tabs-panel
@include tabs-panel;
Adds styles for an individual tab content panel within the tab content container.
JavaScript Reference
Initializing
The following files must be included in your JavaScript to use this plugin:
foundation.core.js
-
foundation.tabs.js
- With utility library
foundation.util.keyboard.js
- With utility library
foundation.util.imageLoader.js
- With utility library
Foundation.Tabs
Creates a new instance of tabs.
var elem = new Foundation.Tabs(element, options);
Fires these events: Tabs#event:init
Name | Type | Description |
---|---|---|
element |
jQuery | jQuery object to make into tabs. |
options |
Object | Overrides to the default plugin settings. |
Plugin Options
Use these options to customize an instance of Tabs. Plugin options can be set as individual data attributes, one combined data-options
attribute, or as an object passed to the plugin's constructor. Learn more about how JavaScript plugins are initialized.
Name | Type | Default | Description |
---|---|---|---|
data-deep-link |
boolean |
false |
Link the location hash to the active pane. Set the location hash when the active pane changes, and open the corresponding pane when the location changes. |
data-deep-link-smudge |
boolean |
false |
If `deepLink` is enabled, adjust the deep link scroll to make sure the top of the tab panel is visible |
data-deep-link-smudge-delay |
number |
300 |
If `deepLinkSmudge` is enabled, animation time (ms) for the deep link adjustment |
data-deep-link-smudge-offset |
number |
0 |
If `deepLinkSmudge` is enabled, animation offset from the top for the deep link adjustment |
data-update-history |
boolean |
false |
If `deepLink` is enabled, update the browser history with the open tab |
data-auto-focus |
boolean |
false |
Allows the window to scroll to content of active pane on load. Not recommended if more than one tab panel per page. |
data-wrap-on-keys |
boolean |
true |
Allows keyboard input to 'wrap' around the tab links. |
data-match-height |
boolean |
false |
Allows the tab content panes to match heights if set to true. |
data-active-collapse |
boolean |
false |
Allows active tabs to collapse when clicked. |
data-link-class |
string |
tabs-title |
Class applied to `li`'s in tab link list. |
data-link-active-class |
string |
is-active |
Class applied to the active `li` in tab link list. |
data-panel-class |
string |
tabs-panel |
Class applied to the content containers. |
data-panel-active-class |
string |
is-active |
Class applied to the active content container. |
Events
These events will fire from any element with a Tabs plugin attached.
Name | Description |
---|---|
deeplink.zf.tabs |
Fires when the plugin has deeplinked at pageload |
change.zf.tabs |
Fires when the plugin has successfully changed tabs. |
collapse.zf.tabs |
Fires when the plugin has successfully collapsed tabs. |
Methods
_handleTabChange
$('#element').foundation('_handleTabChange', $target, historyHandled);
Opens the tab $targetContent
defined by $target
. Collapses active tab.
Fires these events: Tabs#event:change
Name | Type | Description |
---|---|---|
$target |
jQuery | Tab to open. |
historyHandled |
boolean | browser has already handled a history update |
_openTab
$('#element').foundation('_openTab', $target);
Opens the tab $targetContent
defined by $target
.
Name | Type | Description |
---|---|---|
$target |
jQuery | Tab to open. |
_collapseTab
$('#element').foundation('_collapseTab', $target);
Collapses $targetContent
defined by $target
.
Name | Type | Description |
---|---|---|
$target |
jQuery | Tab to collapse. |
_collapse
$('#element').foundation('_collapse');
Collapses the active Tab.
Fires these events: Tabs#event:collapse
selectTab
$('#element').foundation('selectTab', elem, historyHandled);
Public method for selecting a content pane to display.
Name | Type | Description |
---|---|---|
elem |
jQuery | jQuery object or string of the id of the pane to display. |
historyHandled |
boolean | browser has already handled a history update |
_destroy
$('#element').foundation('_destroy');
Destroys an instance of tabs.
Fires these events: Tabs#event:destroyed