Toggler
JavaScript Motion UIToggler makes it easy to toggle CSS or animate any element with a click.
Toggle a CSS class
To setup a class toggle, start by adding the attribute data-toggler
to an element. The value of data-toggler
is the class you want to toggle (like .classname
and classname
). Also give the element a unique ID so it can be targeted.
<ul class="menu" id="menuBar" data-toggler=".expanded">
<li><a href="#">One</a></li>
<li><a href="#">Two</a></li>
<li><a href="#">Three</a></li>
<li><a href="#">Four</a></li>
</ul>
Then, add data-toggle
to any element, with the ID of the target as the value of the attribute. Now, any time you click on this element, the class will toggle on and off on the target.
<p><a data-toggle="menuBar">Expand!</a></p>
Toggle with Animation
Instead of toggling a class, you can also toggle visibility. When toggled, the element comes into or out of view using a Motion UI class.
Instead of data-toggler
, add the attribute data-animate
. The value of the attribute is the in animation you want, followed by the out animation.
<p><a data-toggle="panel">Toggle Panel</a></p>
<div class="callout" id="panel" data-toggler data-animate="hinge-in-from-top spin-out">
<h4>Hello!</h4>
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Dicta quas optio alias voluptas nobis iusto mollitia asperiores incidunt reprehenderit doloribus voluptatibus officiis minus, inventore, quasi nisi. Consequuntur, quidem. Sint, dicta?</p>
</div>
Hello!
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Dicta quas optio alias voluptas nobis iusto mollitia asperiores incidunt reprehenderit doloribus voluptatibus officiis minus, inventore, quasi nisi. Consequuntur, quidem. Sint, dicta?
Mark as Closable
To create an element that can be closed once, add the attribute data-closable
. Then add a click trigger inside the element using data-close
.
<div class="callout" data-closable>
<button class="close-button" data-close>×</button>
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Labore praesentium sint alias dolorum qui vel quaerat, libero consequatur non esse asperiores veritatis commodi, odit eum ipsam nemo dicta iste aliquam.</p>
</div>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Labore praesentium sint alias dolorum qui vel quaerat, libero consequatur non esse asperiores veritatis commodi, odit eum ipsam nemo dicta iste aliquam.
With Alternate Animation
data-closable
can be configured with a custom exit animation.
<div class="callout" data-closable="slide-out-right">
<button class="close-button" data-close>×</button>
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Labore praesentium sint alias dolorum qui vel quaerat, libero consequatur non esse asperiores veritatis commodi, odit eum ipsam nemo dicta iste aliquam.</p>
</div>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Labore praesentium sint alias dolorum qui vel quaerat, libero consequatur non esse asperiores veritatis commodi, odit eum ipsam nemo dicta iste aliquam.
Toggle on focus
The data-toggle
attribute only toggles classes/visibility on click. You can also have the toggle fire when an element is focused or unfocused using data-toggle-focus
.
<input type="text" data-toggle-focus="form-callout" placeholder="Click in here to reveal extra content">
<div class="secondary callout is-hidden" id="form-callout" data-toggler="is-hidden">
<p>This is only visible when the above field has focus.</p>
</div>
This is only visible when the above field has focus.
Multiple Targets
The data-toggle
, data-close
, and data-open
attributes can now target multiple elements! The syntax is simple; just pass a space separated list to the data-x
attribute like so:
<button class="button" data-toggle="foo bar baz">Toggle things</button>
Then the elements with ids of foo
, bar
, and baz
will be toggled any time your button, (or any other element you choose), is clicked.
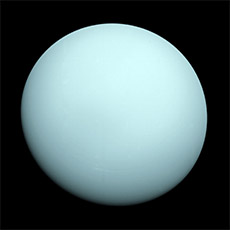
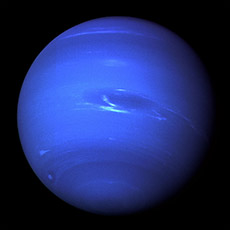
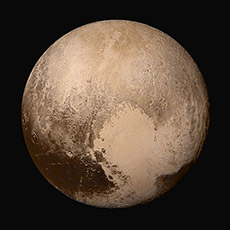
JavaScript Reference
Initializing
The following files must be included in your JavaScript to use this plugin:
foundation.core.js
-
foundation.toggler.js
- With utility library
foundation.util.motion.js
- With utility library
foundation.util.triggers.js
- With utility library
Foundation.Toggler
Creates a new instance of Toggler.
var elem = new Foundation.Toggler(element, options);
Fires these events: Toggler#event:init
Name | Type | Description |
---|---|---|
element |
Object | jQuery object to add the trigger to. |
options |
Object | Overrides to the default plugin settings. |
Plugin Options
Use these options to customize an instance of Toggler. Plugin options can be set as individual data attributes, one combined data-options
attribute, or as an object passed to the plugin's constructor. Learn more about how JavaScript plugins are initialized.
Name | Type | Default | Description |
---|---|---|---|
data-toggler |
string |
|
Class of the element to toggle. It can be provided with or without "." |
data-animate |
boolean |
false |
Tells the plugin if the element should animated when toggled. |
Events
These events will fire from any element with a Toggler plugin attached.
Name | Description |
---|---|
on.zf.toggler |
Fires if the target element has the class after a toggle. |
off.zf.toggler |
Fires if the target element does not have the class after a toggle. |
Methods
toggle
$('#element').foundation('toggle');
Toggles the target class on the target element. An event is fired from the original trigger depending on if the resultant state was "on" or "off".
Fires these events: Toggler#event:on Toggler#event:off
_destroy
$('#element').foundation('_destroy');
Destroys the instance of Toggler on the element.